AP CSP Pseudocode Review (CSP4)
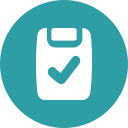
Assessment
•
Phillip Sanborn
•
Computers
•
11th - 12th Grade
•
1 plays
•
Medium
Improve your activity
Higher order questions
Match
•
Reorder
•
Categorization
actions
Add similar questions
Add answer explanations
Translate quiz
Tag questions with standards
More options
31 questions
Show answers
1.
Multiple Choice
After running the following code segment, what is contained in the array data? (array a.k.a list)
2.
Multiple Choice
Choose the best description of what the mystery procedure below does. The procedure accepts two parameters: a list of a values and a number n.
PROCEDURE mystery (list, n)
{
i = 1
REPEAT LENGTH(list) TIMES
{
IF ( list[i] = n )
{
DISPLAY (i)
}
i = i+1
}
}
Mystery displays the number at index n.
Mystery displays the index of the first occurrence of the value n in the list.
Mystery displays the index of the last occurrence of the value n in the list.
Mystery displays the index of every occurrence of the value n in the list.
Mystery displays true if n is in the list.
3.
Multiple Choice
Which of the following best describes the value returned by the procedure below?
PROCEDURE mystery (data)
{
count = 0
i = 1
REPEAT UNTIL (i = LENGTH(data))
{
IF (data[i] < data[i+1])
{
count = count + 1
}
i = i+1
}
RETURN (count)
}
The answers below refer to values being in ascending and descending order. Ascending order means increasing, as in: [1, 2, 5, 8]. Descending order means decreasing, as in [20, 15, 7, 3].
The procedure returns true when data is in ascending order
The procedure returns false when data is in descending order
The procedure returns the number of times adjacent items are in ascending order
The procedure returns how long it takes to find two numbers in ascending order
The procedure counts how long it takes to find two numbers in descending order and returns that number
4.
Multiple Choice
Consider the playGame procedure below which calls on 3 other procedures: countFives, player1Move, and player2Move.
PROCEDURE playGame()
{
cards = []
REPEAT_UNTIL ( countFives(cards) >= 5 )
{
card1 = player1Move()
APPEND (cards, card1)
card2 = player2Move()
APPEND (cards, card2)
}
}
The procedure above simulates a certain card game called "fives" - played with two decks of cards - in which each player takes a turn playing a card, until 5 fives have been played in total, at which point it's "Game Over." The procedure uses a list called cards which is initially empty. Each round of play, two cards are appended to the list.
Here is the countFives procedure.
PROCEDURE countFives(cards)
{
count = 0
FOR EACH card IN cards
{
IF( card = 5 )
{
count = count+1
}
}
<MISSING CODE>
}
Which of the following should replace the <MISSING CODE> at line 12 to make the procedure work as designed?
DISPLAY (count)
DISPLAY ("game over")
RETURN (count)
RETURN ("game over")
Nothing. Procedure works as is.
5.
Multiple Choice
A robot starts in a grid facing north (B3). What is the robot's location and direction after the following program is executed?
data = ["F", "F", "R", "F", "L", "F", "R", "R", "F"]
FOR EACH move IN data
{
IF ( move = "F" AND CAN_MOVE(forward) )
{
MOVE_FORWARD()
}
ELSE IF (move = "R"){
rotate_right()
}
ELSE IF ( move = "L") {
rotate_left()
}
}
6.
Multiple Choice
Which of the following best describes the result of running the procedure below?
PROCEDURE mystery (a, b, c)
{
IF ( a >= b AND a >= c)
{
RETURN (a)
}
ELSE IF ( b >= a AND b >= c)
{
RETURN (b)
}
ELSE
{
RETURN (c)
}
}
mystery returns the smallest of the three input values
It returns the middle of the three input values
It returns the largest of the three input values
It returns the average of the three input values
It always returns c
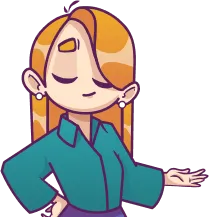
Explore this activity with a free account
Find a similar activity
Create activity tailored to your needs using
Computer Basics
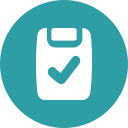
•
6th - 8th Grade
Web Design
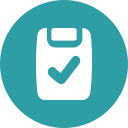
•
6th - 9th Grade
While Loops
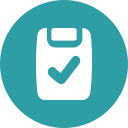
•
9th - 12th Grade
CodeCombat
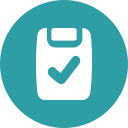
•
8th Grade
CodeHS JavaScript
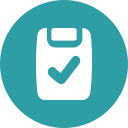
•
8th - 12th Grade
Web Design
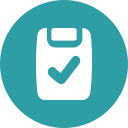
•
10th - 12th Grade
Integer Operations
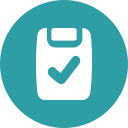
•
6th Grade
Web Development Review
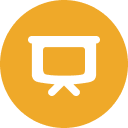
•
9th - 12th Grade